OOPS Concepts-JAVA
Java OOPs (Object-Oriented Programming) Concepts(oo)
Note: This blog is just to share our Knowledge if there are any mistakes please let us know.
What is oo programming..?
OO has strong historical roots in other
paradigms and practices. It came about to address problems commonly grouped
together as the “software crisis.”
It does not solve any questions its just a piece of
solution for problems.
OOPs Concept in Java
· Polymorphism
·
Inheritance
·
Encapsulation
·
Abstraction
·
Class
·
Object
·
Method
·
Message Passing
Note: We are going to explain these concepts in Java
programming.
1)Polymorphism:
POLYMORPHIC: MANY FORMS.
Polymorphism is something (function argument, variable, etc)
can be of more than one type.
Not limited to OO languages.If we take
concept of operater overloading. For example in C, we have operators like +, -,
*, etc, which operate for all primitive types.
In other words we don’t have separate ”+ int”, ”+ float”, ”+ char”
etc, operators for each type.So we can call “+ “as polymorphic.
In Java Polymorphism achieved by following:
1.
Overloading
2.
Overriding
Method overloading
means having two or
more methods with the same name but different signatures in the same scope.
These two methods may exist in the same class or another one in base class and
another in derived class,It is compile time execution(polymorphism) or early
binding.
class Person { private String firstName; private String lastName; Person() { this.firstName = ""; this.lastName = ""; } Person(String FirstName) { this.firstName = FirstName; this.lastName = ""; } Person(String FirstName, String LastName) { this.firstName = FirstName; this.lastName = LastName; } }
Calling Overloaded
Methods.
Person(); // as a
constructor and call method without parameter
Person(userFirstName);
// as a constructor and call method with one parameter
Person(userFirstName,userLastName);
// as a constructor and call method with one parameter
When to use Method
Overloading?
Generally, you
should consider overloading a method when you have required same reason that
take different signatures, but conceptually do the same thing.
Method Overriding :
means having a
different implementation of the same method in the inherited class. These two
methods would have the same signature, but different implementation. One of
these would exist in the base class and another in the derived class. These
cannot exist in the same class.
Step 1: In this
example we will define a base class called Circle
class Circle { protected double radius; public Circle(double radius) { this.radius = radius; } public double getArea() { return Math.PI*radius*radius; } }
When the getArea
method is invoked from an instance of the Circle class, the method returns the
area of the circle.
Step 2
The next step is to
define a subclass to override the getArea() method in the Circle class. The
derived class will be the Cylinder class. The getArea() method in the Circle
class computes the area of a circle, while the getArea method in the Cylinder
class computes the surface area of a cylinder.
The Cylinder class
is defined below.
class Cylinder extends Circle { protected double length; public Cylinder(double radius, double length) { super(radius); this.length = length; } public double getArea() { // method overriden here return 2*super.getArea()+2*Math.PI*radius*length; } }
When the overriden
method (getArea) is invoked for an object of the Cylinder class, the new
definition of the method is called and not the old definition from the
superclass(Circle).
Example code
This is the code to
instantiate the above two classes
Circle myCircle;
myCircle = new
Circle(1.20);
Cylinder
myCylinder;
myCylinder = new
Cylinder(1.20,2.50);
2)Inheritance:
·
The concept of getting properties of one class object to another
class object is known as inheritance.
·
Here properties means variable and methods.
Types of Inheritance:
1. Multiple
inheritance.
2. Multilevel
inheritance.
Multilevel Inheritance:
·
Getting the properties from one class object to another class
object level wise with some priority is known as multilevel inheritance.
class A{
}
class B
extends A{
}
class C
extends B{
}
Multiple inheritance:
·
The concept of Getting the properties from multiple class objects
to sub class object with same priorities is known as multiple inheritance.
·
Java Doesn't Support multiple Inheritance.
·
multiple inheritance achieve by using interfaces
/Multiple
inheritance program
interface A{ public void show(); } interface B{ public void display(); } Class C Implements A,B{ }
3)Encapsulation:
Simply,
encapsulation means the bringing together of a set of attributes and methods
into an object definition and hiding their implementational structure from the
object's users.
Therefore,
how an object structures and implements its attributes and methods is not
visible to other objects using it. Direct access to an object's attributes is
not permitted and any changes to the object's data can only be effected
indirectly via a set of publicly available methods.
Why encapsulate and hide?
• You can delay the resolution of the details until after the
design.
• You can change it later without a ripple effect.
• It keeps your code modular.
How we do this separation is with two separate pieces for each
class, an implementation and an interface.
Basic java example program on data encapsulation
public class EncapsulationDemo { String name; int rno; String address; public String getName() { return name; } public void setName(String name) { this.name = name; } public int getRno() { return rno; } public void setRno(int rno) { this.rno = rno; } public String getAddress() { return address; } public void setAddress(String address) { this.address = address; } public void showInfo(){ System.out.println("Name: "+getName()); System.out.println("R.No: "+getRno()); System.out.println("Name: "+getAddress()); } }
4) Abstraction
Humans
deal with complexity by abstracting details away.
Abstraction is used to
hide certain details from the user that they might find irrelevant
Examples:
• Driving
a car doesn’t require knowledge of internal combustion engine; sufficient to
think of a car as simple transport.
• Stereotypes are negative examples of
abstraction.
To be useful, an abstraction (model) must be
smaller than what it represents. (road map vs photographs of terrain vs
physical model).
Difference between
Abstraction and Encapsulation
Abstraction |
Encapsulation |
Abstraction
solves the issues at the design level. |
Encapsulation
solves it implementation level. |
Abstraction
is about hiding unwanted details while showing most essential information. |
Encapsulation
means binding the code and data into a single unit. |
Abstraction
allows focussing on what the information object must contain |
Encapsulation
means hiding the internal details or mechanics of how an object does
something for security reasons. |
The above are
the main 4 oops concepts.
5) Message Passing:
Definition: Message passing is the procedure through
which objects communicate with each other bypassing the reliable information to
run the program such as a request for the execution of the function or request
to pass the values.
The message includes the information of the information to be
sent and the name of the object or function.
6 )Class:
Definition: A class is a user-defined entity through
which the objects are created. It represents a collection of properties or
methods that is common to every object of a similar kind.
7) Objects:
Definition: It is the basic unit of the
Object-Oriented Programming(OOPs) it is used to represent the real-life entity.
Any typical java program includes many objects, and these objects interact with
each other through the invoking method.
8) Method:
Definition: A method is a collection of statements
that are used to perform the specific task and it returns the obtained value to
the caller. A method is also capable of performing a specific task without
returning any values. In Java, unlike other languages, a method should be a
part of the class.
Conclusion:
These are
the important oops concept which are helped
to design a solution to problems
This not oops concept I like this visulazation and i like to share with you
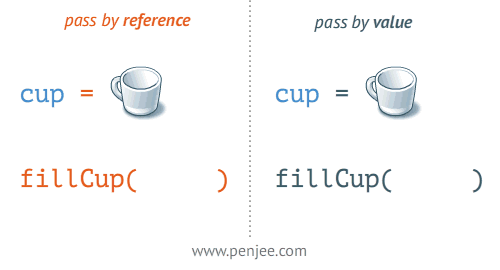
Any Quires
Comment below
Note: This blog is just to share our Knowledge if there are any mistakes please let us know.
Thanks.
Nice
ReplyDelete